Reverse Iterator C++
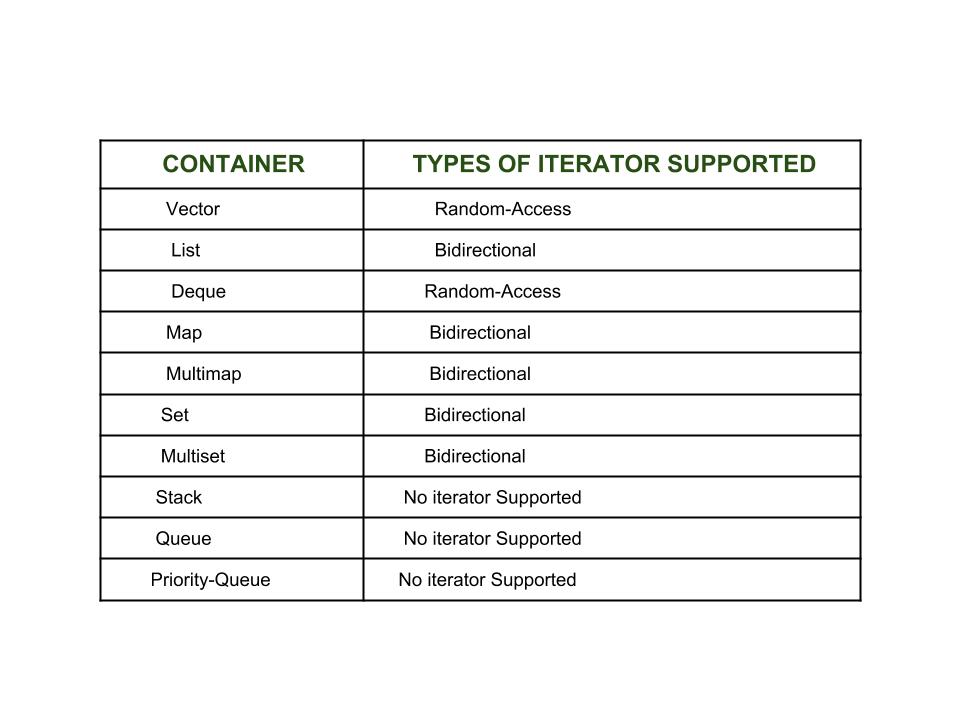
Introduction To Iterators In C Geeksforgeeks
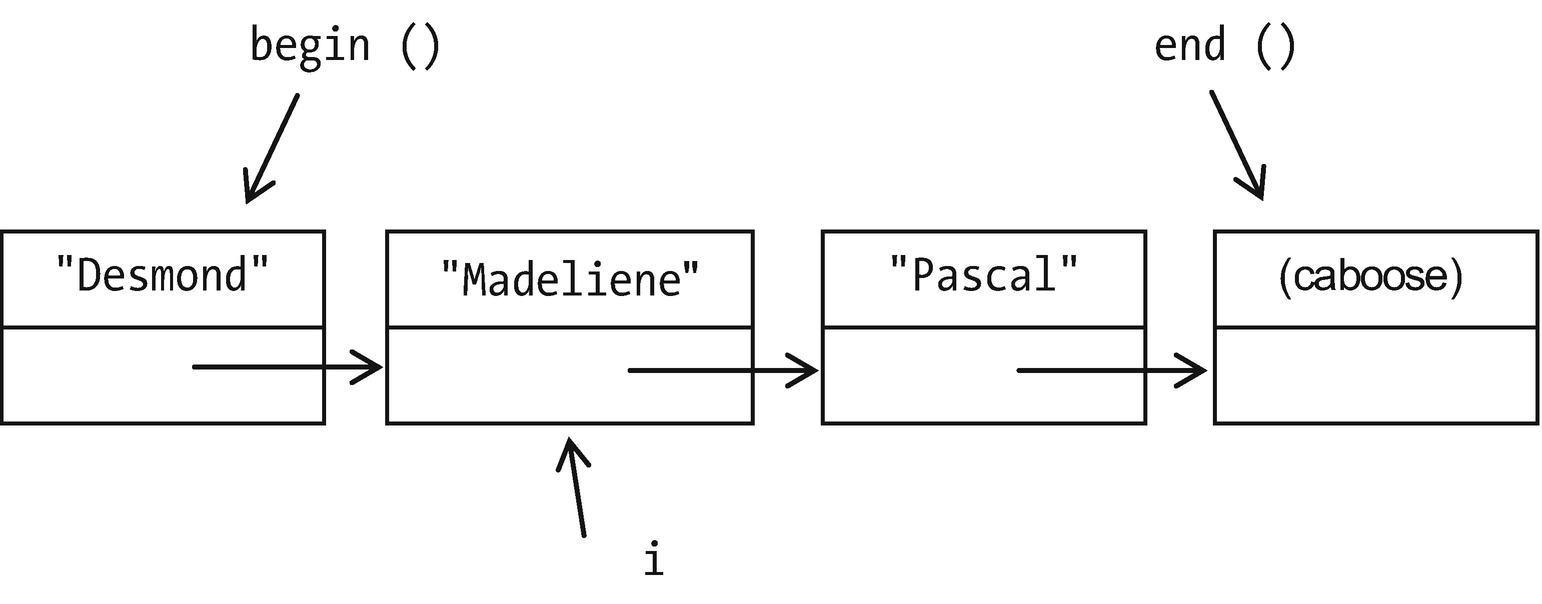
The Standard Template Library Springerlink
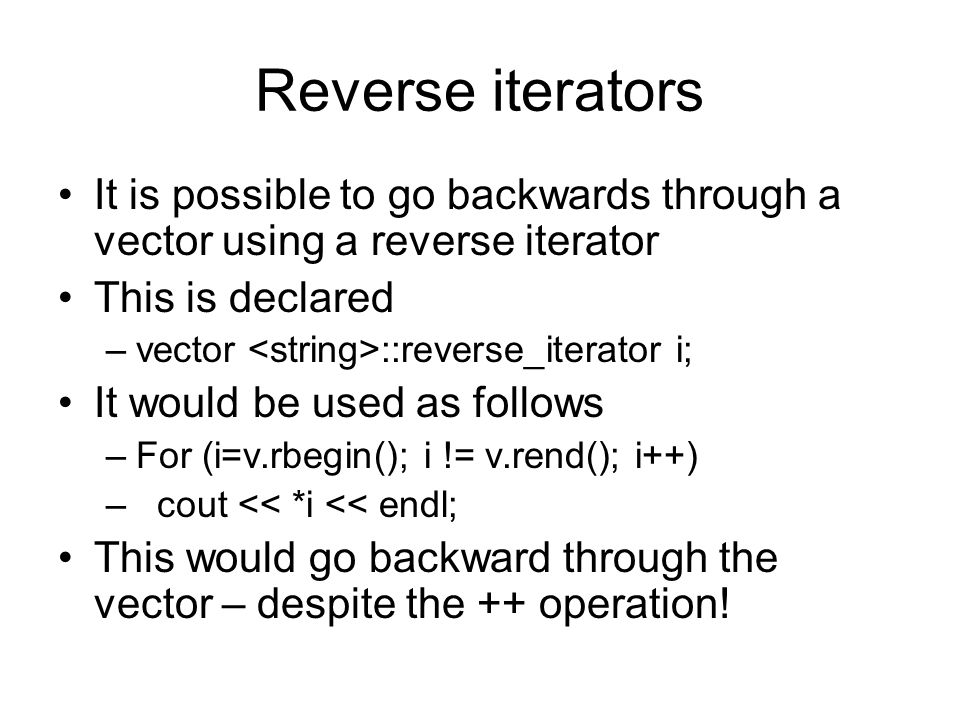
Vectors Lists And Queues Ppt Video Online Download

C Code Snippet Of Our Cpu Implementation Of Gray Pattern Decoder Download Scientific Diagram
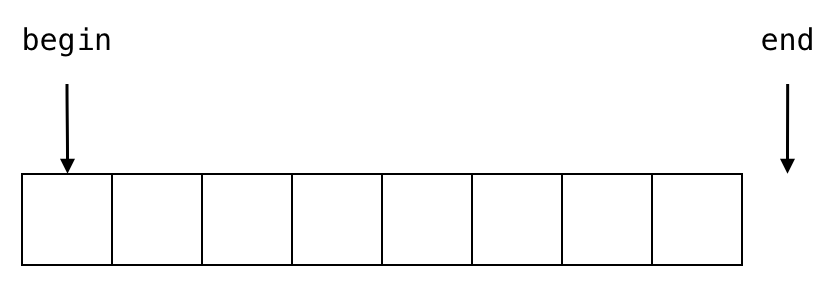
Iterators Data Structures
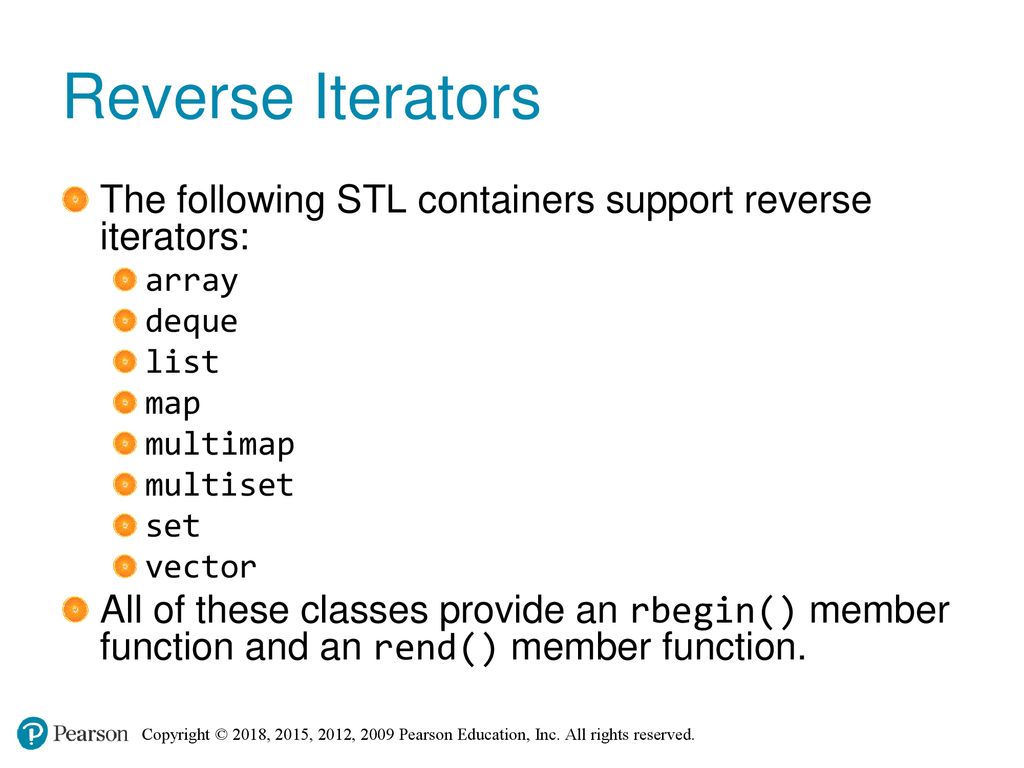
The Standard Template Library Ppt Download
Corrects many of the shortcomings of C++98's std::reverse_iterator.
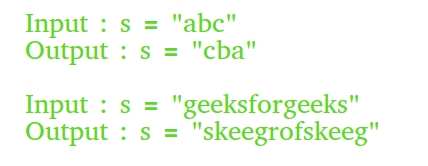
Reverse iterator c++. This iteration will not change the order of the list and we will not do any modification to the list elements. To understand this example, you should have the knowledge of the following C++ programming topics:. For this to work properly, we need to change the return type of begin() and end() to auto, so that we get const_reverse_iterator back from them.
For example, the given number is 864, then the reverse of this number will be 468. The initial question was to compare std::copy_backward with using reverse iterators. Vector::rend() is a built-in function in C++ STL which returns a reverse iterator pointing to the theoretical element right before the first element in the array container.
In addition, it may model old iterator concepts specified in the following table:. Program to demonstrate the set::rbegin() method:. This problem is solved by using while loop in this example.
When Iterator is vector<int>::iterator, if that happens to be int *, then --ri.base() must fail to compile (because int * is not of class type). Why does this range loop decrease FPS by 35?. Where, rbegin() stands for reverse beginning.
The similarity between these last two code examples comes from a similarity between unsigned integers and iterators:. A reverse iterator is made from a bidirectional, or random access iterator which it keeps as a member which can be accessed through base(). For instance, to iterate backwards use:.
The function returns a reverse iterator pointing to the theoretical element right before the first element in the array container. Compiled as C++ Code (/TP) Other info:. Visual studio 19 version 16.5 cppcompiler C++ Fixed In:.
See the very bottom of the post. Returns a reverse iterator pointing to the last element in the container (i.e., its reverse beginning). Constexpr std::reverse_iterator<Iter> make_reverse_iterator( Iter i );.
Iterating over a map will result in above order i.e. Using the C++ rbegin() function to return an iterator to the first element in a reversed string and rend() to return an iterator that points just beyond the last element in a reversed string. Operators for the C++ Standard Template Library Output:.
Please note each of these methods have O(1) complexity:. So it seems easy to reverse the elements of a vector by the help of header file algorithm. This is a quick tutorial on how to use reverse iterator in C++.
Rbegin points to the element right before the one that would be pointed to by member end. Returns a reverse iterator pointing to the last element in the vector (i.e., its reverse beginning). All the STL containers (but not the adapters) define.
(1) I'm writing a game using SFML and C++11 features, such as the range loop. A type that provides a reference to a const element stored in a list for reading and performing const operations. Reversed () function returns an iterator to accesses the given list in the reverse order.
It means printing the given number back to the front. Increasing them moves them towards the beginning of the container. C++ provides two non-const iterators that move in both the directions are iterator and reverse iterator.
If a container supports a random access iterator or a bidirectional iterator then we can traverse it in a reverse order using an iterator. Is usually implemented as a dynamic array by most libraries. Returns a reverse iterator pointing to the reverse beginning of vector.
After the call to std::copy_backward, the collection is in the following state:. Visual Studio 19 version 16.5 Preview 2 Vemund Handeland reported Dec 06, 19 at 02: PM. A function or a function object that returns a boolean (true/false) value or an integer value:.
If you're really stuck with C++11, then we'll have to add the trailing return type:. The iterator types for that container, e.g., iterator and const_iterator, e.g., vector<int>::iterator;. Reverse iterators iterate backwards:.
++it){ cout << *it;} // prints. We have different ways to traverse a list in reverse order. We hope that you have got a clear idea of how to decrement an iterator in C++.
Thus a reverse iterator constructed from a one-past-the-end iterator dereferences to the last element in a sequence. If you are using the LinkedList instead of an ArrayList, you can get the reverse Iterator using the descendingIterator method of the LinkedList class. The function returns a reverse iterator pointing to the last element in the container.
Visual Studio 19 version 16.4.3 Fixed In:. It would be nice to be able to use C++11 range for loops to iterate backwards. Function adaptors and function objects used to reverse the sense of predicate function objects:.
Notice that unlike member vector::back, which returns a reference to this same element, this function returns a reverse random access iterator. Increasing them moves them towards the beginning of the container. Std::vector<int> v{1, 2, 3, 4, 5};for (std::vector<int>::reverse_iterator it = v.rbegin();.
The function does not take any parameter. Converting Between Iterators and Indexes in a Vector. Suppose x and y are the two iterators:.
Here we will easily learn, how to reverse a vector in c++. An iterator which traverses the elements of some bidirectional sequence in reverse. The iterator returned by this method returns list elements in reverse order.
C++ Bidirectional iterator has the same features like the forward iterator, with the only difference is that the bidirectional iterator can also be decremented. Reverse_iterator<Iterator>::base() is required to return Iterator. Iterate over a deque in C++ (Forward and Backward directions) In this post, we will discuss how to iterate over a deque in C++ in forward and backward directions.
Reverse iterators are those which iterate from backwards and move towards the beginning of the deque. For example, if you store following elements in map i.e. It is at the end of the destination collection, since this is where std::copy_backward has to start writing its results.
For example, an array container might offer a forwards iterator that walks through the array in forward order, and a reverse iterator that walks through the array in reverse order. C++ Program to Reverse a Number Example to reverse an integer entered by the user in C++ programming. Reverse_iterator - range based for loop c++.
A deque (or Double ended queue) is sequence container that can be expanded or contracted on both its ends. C++ Iterator Library - reverse_iterator - It is an iterator adaptor that reverses the direction of a given iterator. A type that provides a bidirectional iterator that can read or modify an element in a reversed list.
C++ STL iterator, operator+ add program example. A write-only, forward moving iterator:. Visual C++ Express Edition 05.
So this is std::copy_backward. Project -> your_project_name Properties -> Configuration Properties -> C/C++ -> Advanced -> Compiled As:. I will show you different ways to achieve this.
Return iterator to end of the list. A type that counts the number of elements in a list. How to iterate a map in reverse order – C++.
Notice that unlike member list::back, which returns a reference to this same element, this function returns a reverse bidirectional iterator. In this post, we will discuss various ways to iterate List in reverse order in Java without actually reversing the list. The range based for uses begin () and end () to get iterators and thus simulating this with a wrapper object can achieve the results we require.
Map store the elements in sorted order of keys. To iterate backwards use rbegin()and rend()as the iterators for the end of the collection, and the start of the collection respectively. It is relatively simple to fix this.
Cycle through v in the reverse direction using a reverse_iterator. Traversing a container in reverse order in C++:. Properties Of Bidirectional Iterator.
Note the output iterator:. This whole thing was nonsense. List::rbegin() and list::rend() functions.
Let’s dive into the iterators provided by std:list. Return iterator to beginning of the list. See if iterator is still in the same spot of memory:.
For my own convenience, I'll work with C++17 from here. Iterators are central to the generality and efficiency of the generic algorithms in the STL. Range-for only works forwards.
Moreover, reverse() is a predefined function in the header file algorithm. Let us see how we can build this logic in C++ with some examples. A specialization of reverse_iterator models the same iterator traversal and iterator access concepts modeled by its Iterator argument.
But unfortunately, there is no such reverse range-for:. An iterator over elements of a container whose lifetime is maintained by a shared_ptr stored in the iterator. This example will show you how to iterate to a vector of struct that consists of multiple members.
The class template is an iterator adaptor that describes a reverse iterator object that behaves like a random-access or bidirectional iterator, only in reverse. It enables the backward traversal of a range. An iterator can be incremented or decremented but it cannot modify the content of deque.
The begin/end methods for that container, i.e., begin() and end() (Reverse iterators, returned by rbegin() and rend(), are beyond the. In other words, when provided with a bidirectional iterator, std::reverse_iterator produces a new iterator that moves from the end to the beginning of the sequence defined by the underlying bidirectional iterator. Auto begin()-> decltype(m_container.begin()), for example.
The function does not take any parameter. Sorted order of keys. Though there is no built-in way to use the range based for to reverse iterate;.
Set::rbegin() is a built-in function in C++ STL which returns a reverse iterator pointing to the last element in the container. In this article we will discuss how to iterate a map in reverse order. C++ provides the wide range of iterator with the lists and here I have listed them along with their function.
(since C++17) make_reverse_iterator is a convenience function template that constructs a std::reverse_iterator for the given iterator i (which must be a LegacyBidirectionalIterator) with the type deduced from the type of the argument. Reverse iterators iterate backwards:. A container may provide different kinds of iterators.
Loop through all elements in a vector in reverse order by using rbegn, rend:. Let’s see how to traverse a collection backwards by using a range for loop. How to use the C++ iterator, operator+ to add an offset to an iterator and returns a move_iterator or a reverse_iterator addressing the inserted element at the new offset position in C++ programming.
Because there is an off-the-end value but no off-the-beginning value, both unsigned integers and iterators require special care when a value might run off the beginning of a range (i.e., might underflow). The List interface provides a special iterator, called a ListIterator, that allows bidirectional access.We can call List.listIterator(index) method to get a ListIterator over the elements in a list starting from specified position in the list. List reverse iterator declaration:.
Overview of Reverse Number in C++. In this tutorial, we will learn how to iterate over a list in reverse order. Rbegin points to the element right before the one that would be pointed to by member end.
C++ Deque rbegin() function returns a reverse iterator pointing to the last element of the container. For a reverse iterator r constructed from an iterator i, the relationship & * r == & * (i-1) is always true (as long as r is dereferenceable);. C++ specification of `std::reverse_iterator` makes creating a `std::string_view` from another `std::string_view`'s reverse iterators impossible.
In this article, we are going to see how we can reverse a number in the C++ language. To iterate a list in reverse order in C++ STL, we need an reverse_iterator that should be initialized with the last element of the list, as a reverse order and we need to check it till the end of the list. When working on tile maps, I basically made a class for each map tile, a light-weight class that simply contains its sprite, position, and such, and then built some.
Firstly, we will store the numbers in a vector and we will create an iterator for it.
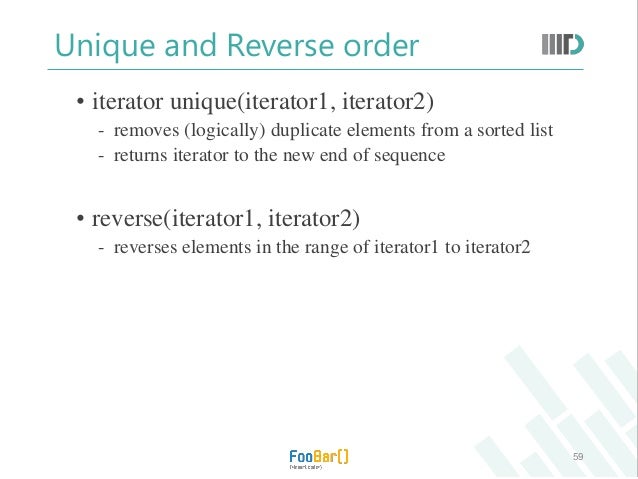
Stl In C

A Gentle Introduction To Iterators In C And Python By Ciaran Cooney Towards Data Science

Llvm Llvm Sys Path Reverse Iterator Class Reference
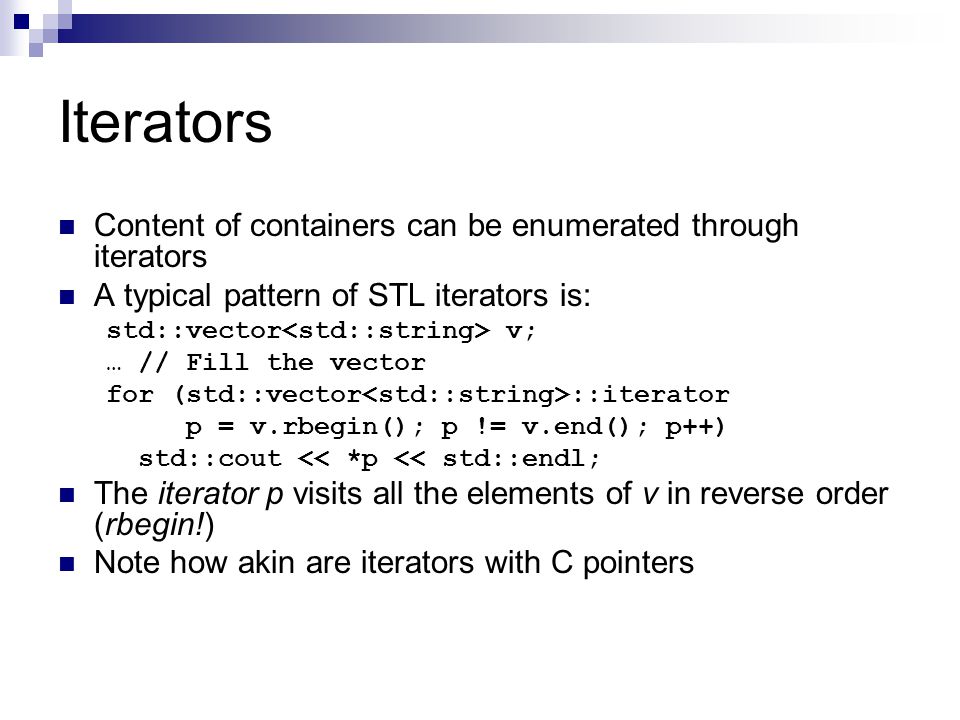
Stl Antonio Cisternino Introduction The C Standard Template Library Stl Has Become Part Of C Standard The Main Author Of Stl Is Alexander Stephanov Ppt Download
Simplified C Stl Lists
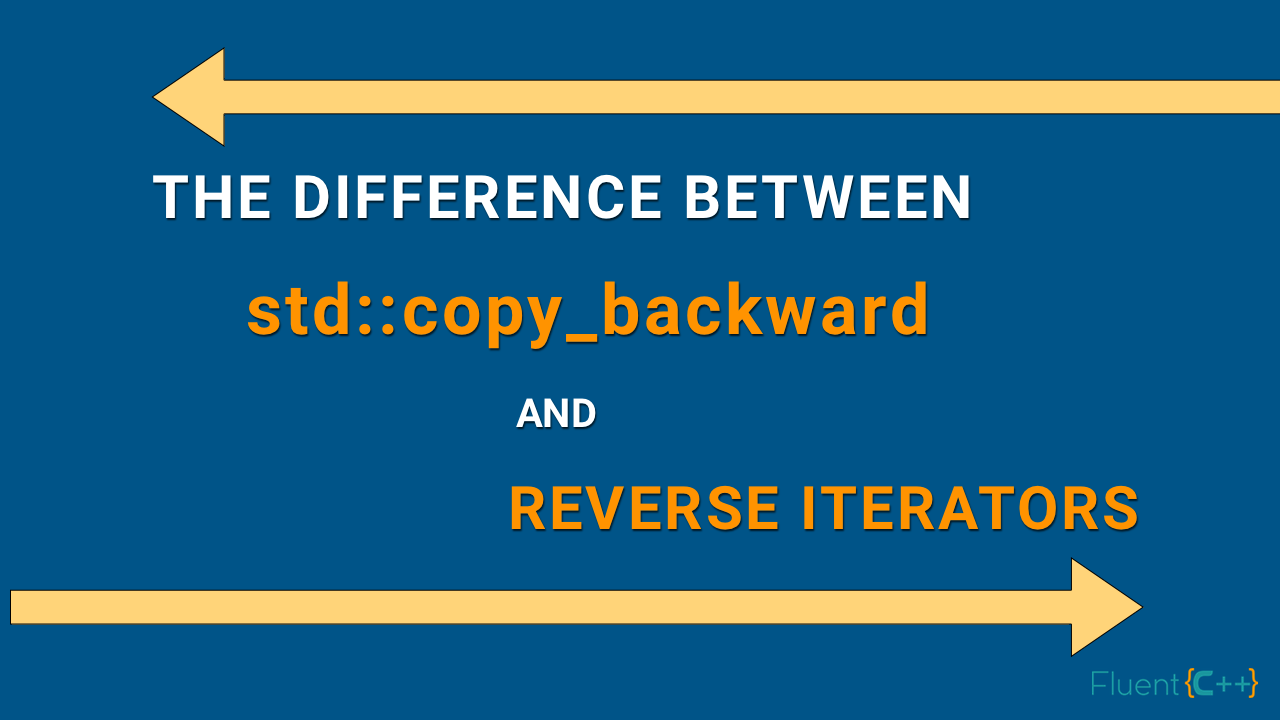
The Difference Between Std Copy Backward And Std Copy With Reverse Iterators Fluent C
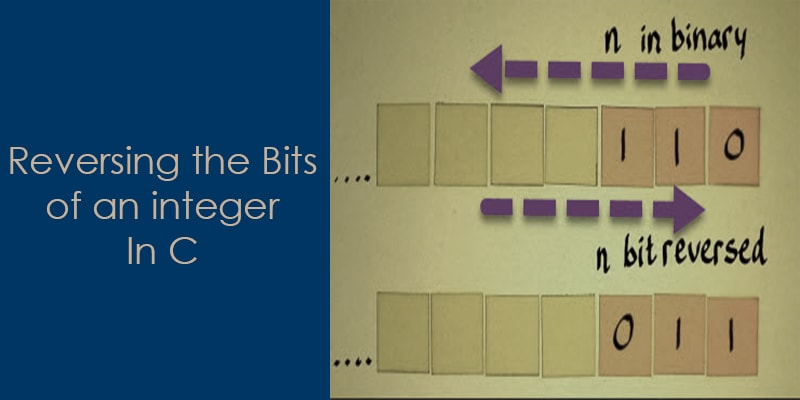
5 Way To Reverse Bits Of An Integer Aticleworld

How To Use Useful Library Stl In C By Anna Kim Medium

Double Ended Queue Deque In C With Examples
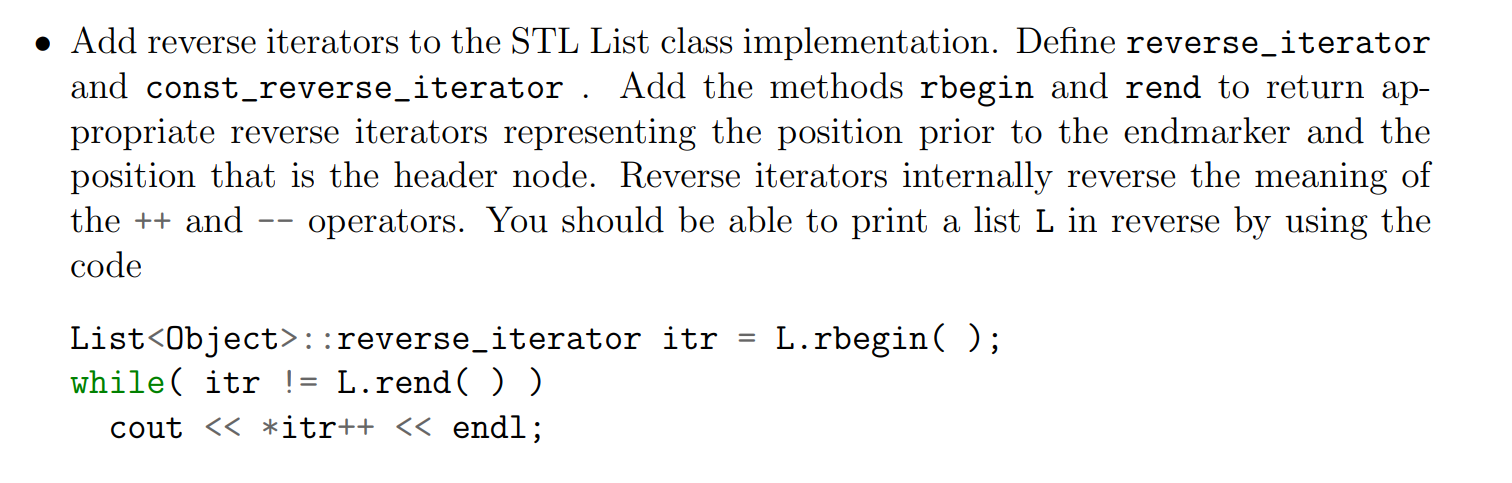
Solved Really Having Troubling Implementing This In C Chegg Com
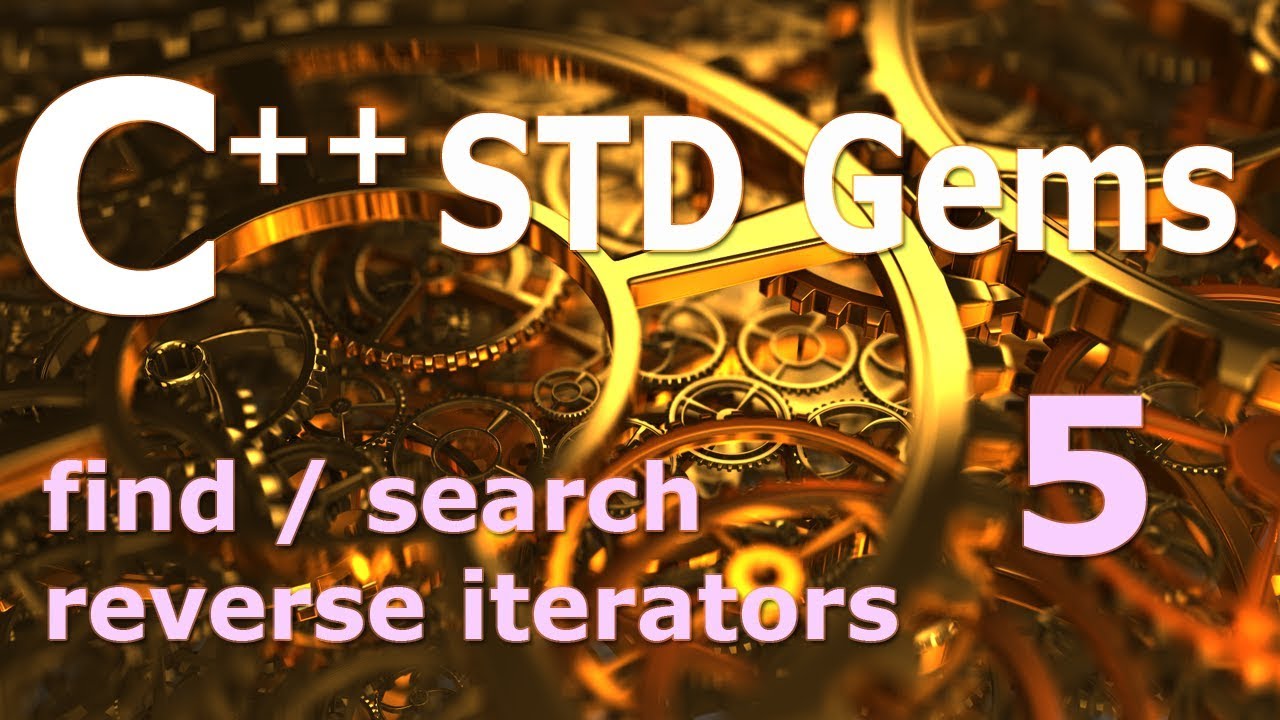
C Std Gems Find Search Reverse Iterators Youtube
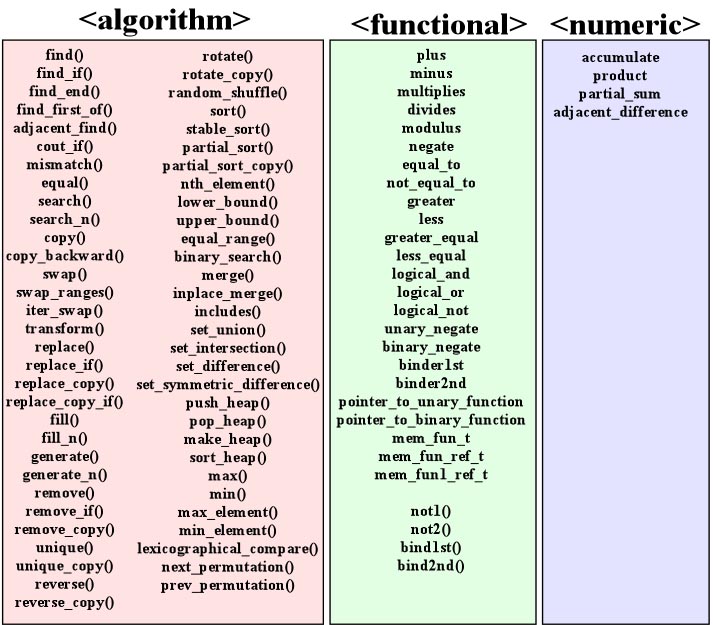
Cs 307
Use Reverse Iterators Deque Data Structure C
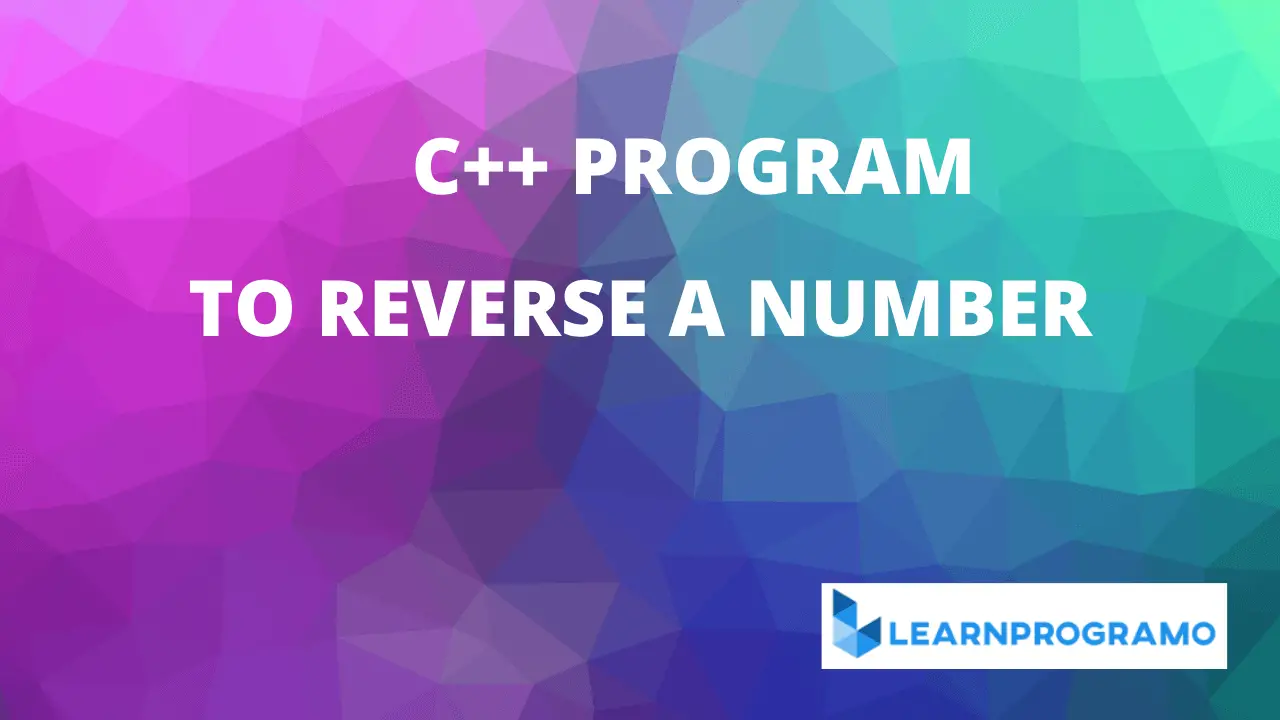
C Program To Reverse A Number With Or Without Using Loop
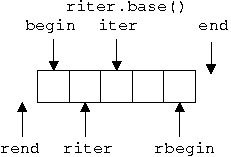
Stl Iterators

Reverse A Number In C Programming Pseudocode Example C Programming Example

Libstdc V3 Source Templatestd Vector Type Alloc Class Reference

Iterator Pattern Wikipedia

Iterator Pattern Wikipedia

How To Call Erase With A Reverse Iterator

Reverse String In C Journaldev
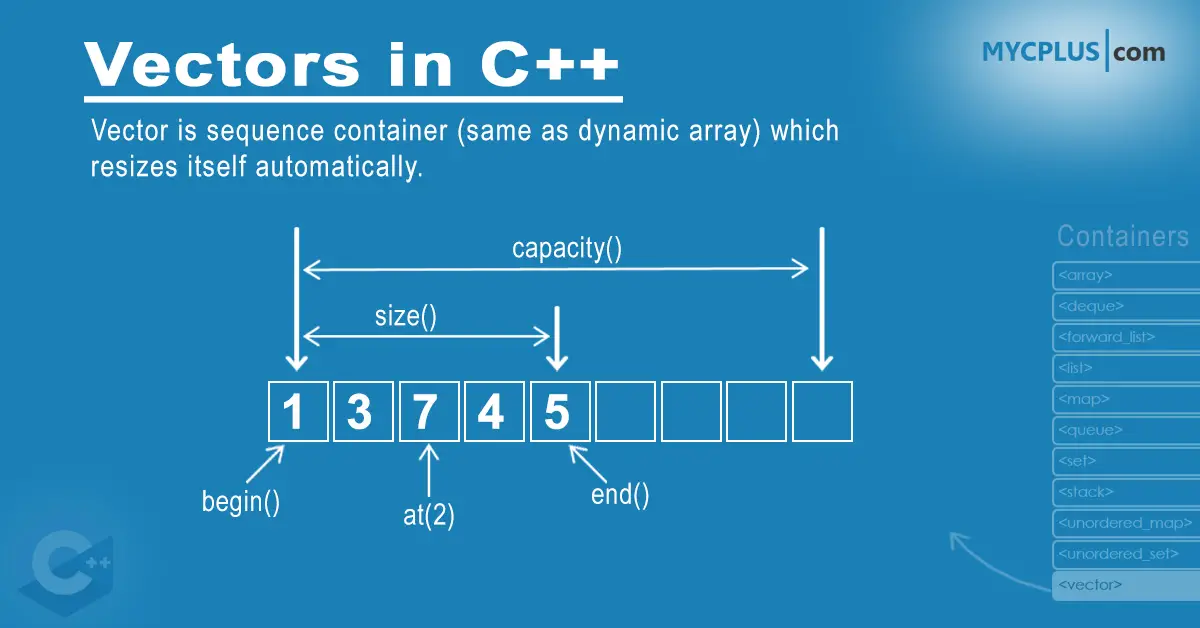
C Vectors Std Vector Containers Library Mycplus
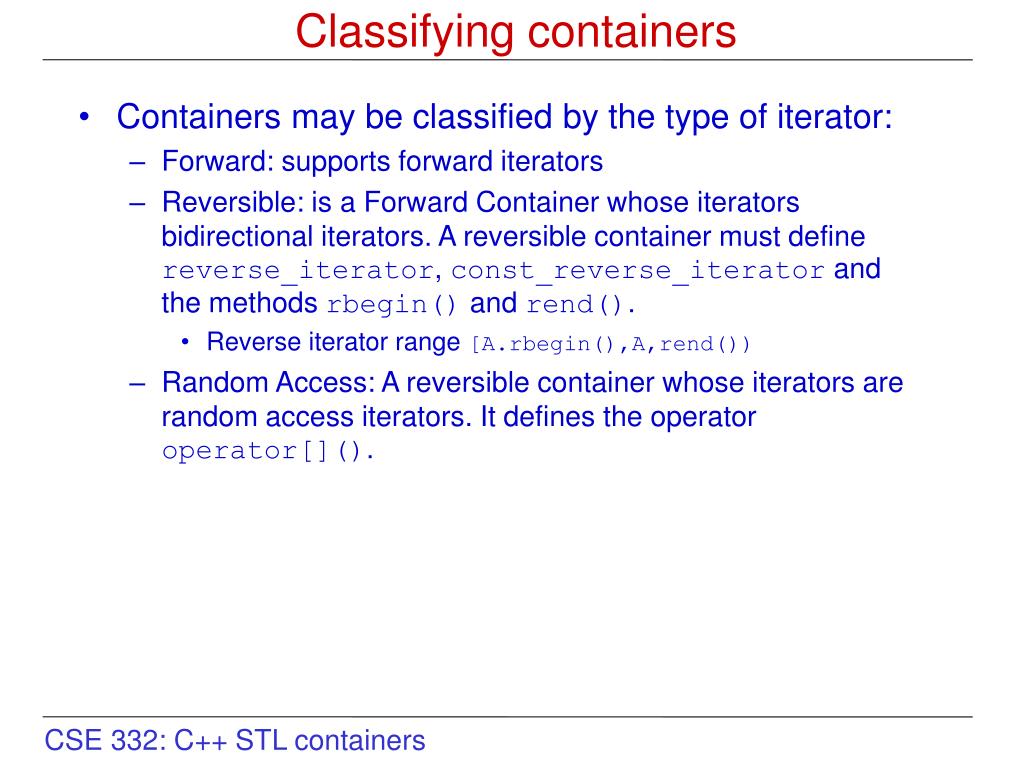
Ppt Intro To The C Standard Template Library Stl Powerpoint Presentation Id

Qmapiterator Class Qt 4 8

Learn Stl Tricky Reverse Iterator Youtube

Three Guidelines For Effective Iterator Usage Dr Dobb S
Std Reverse Iterator Cppreference Com
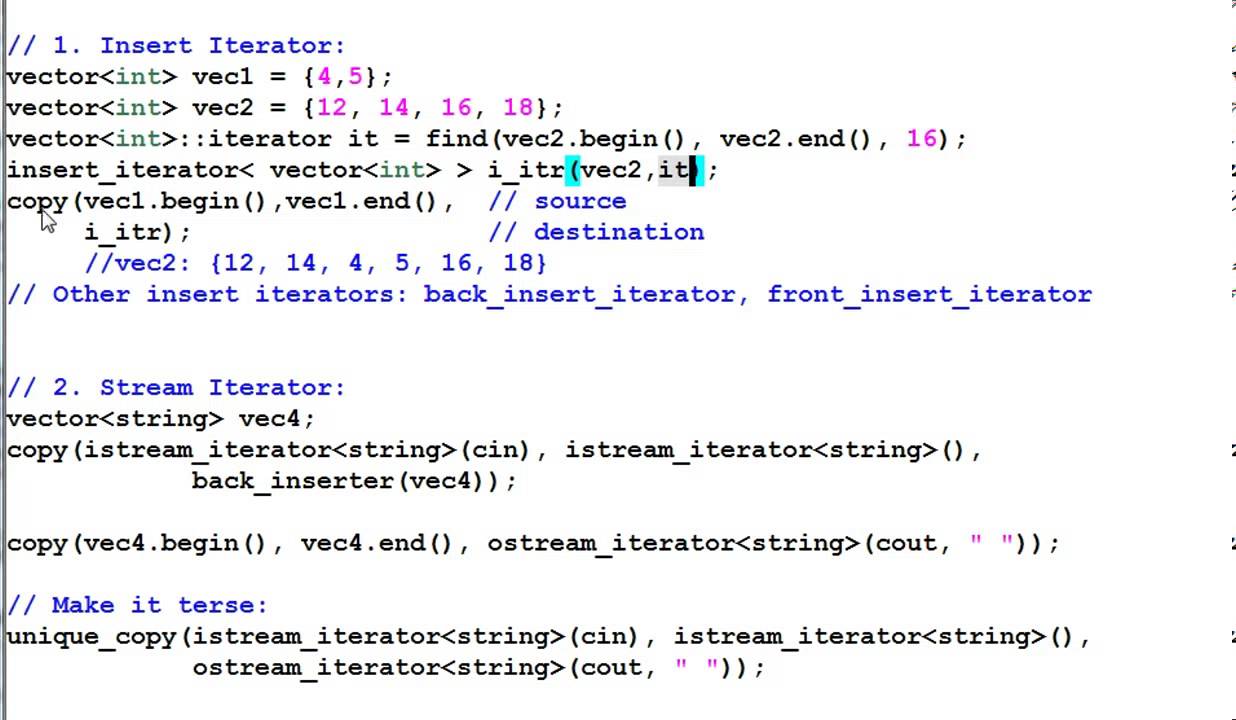
Introduction Of Stl 5 Iterators And Algorithms Youtube

Why Does A Push Back On An Std List Change A Reverse Iterator Initialized With Rbegin Stack Overflow

Understanding Stl Iterators Reverse Iterators Part 1 By Chukwunonso Nwafor Understanding Stl Iterators Medium
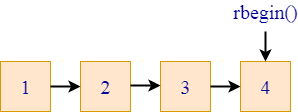
C Deque Rbegin Function Javatpoint
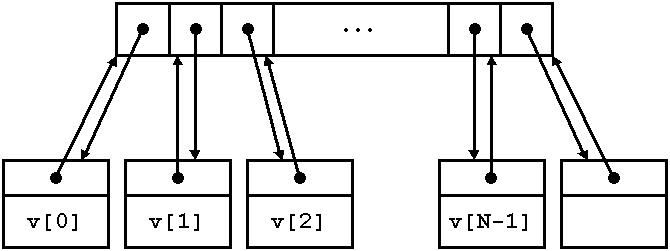
Non Standard Containers 1 60 0
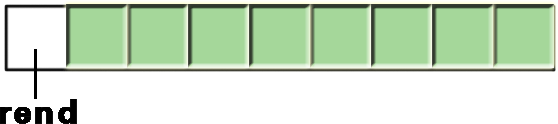
C Std Array Declare Initialize Passing Std Array To Function
C String Rend Function Alphacodingskills
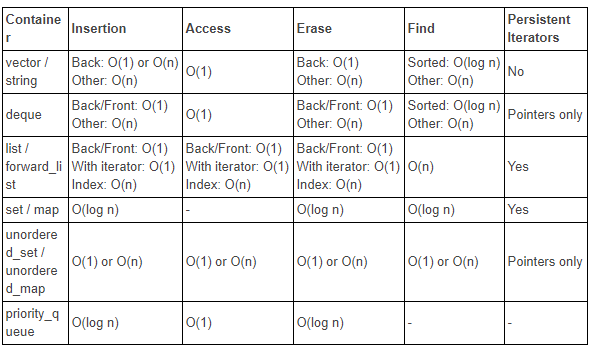
Stl Container Performance Stl Container Performance Table By Onur Uzun Medium
Http Www Aristeia Com Papers Cuj June 01 Pdf

For Each On A Reverse Iterator Of A Custom Bidirectional Iterator Requires Outputiterator Stack Overflow

Iterator Ienumerator In C And C As Function Method Parameters And Arg Reading Writing Data Structures Structure In C
Stl C Why Did The Reverse Iterator For The List Container In C Crash In Vs19

Write A Python Program To Reverse A Number
Www Sciencedirect Com Science Article Pii Sx Pdf Md5 C5f192fac2a124ba10f4c Pid 1 S2 0 Sx Main Pdf
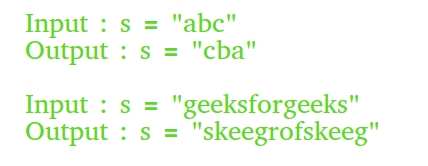
Different Methods To Reverse A String In C C Geeksforgeeks
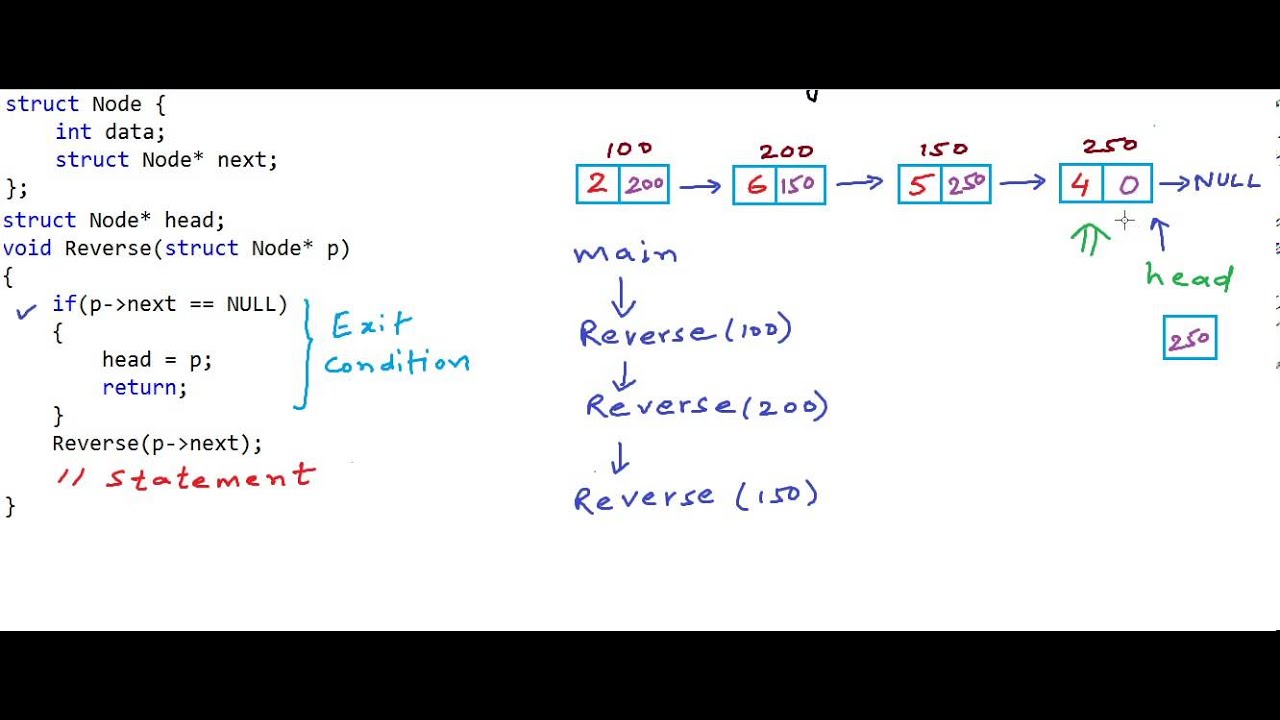
Reverse A Linked List Using Recursion Youtube
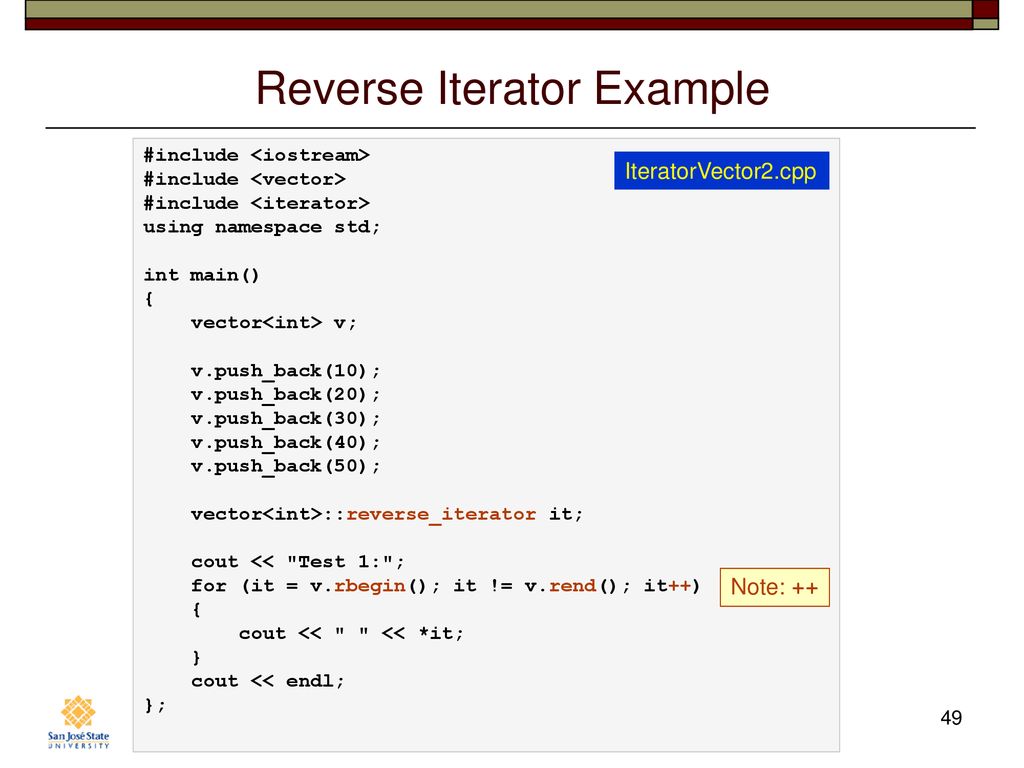
Cmpe Data Structures And Algorithms In C October 19 Class Meeting Ppt Download

Ppt Intro To The C Standard Template Library Stl Powerpoint Presentation Id
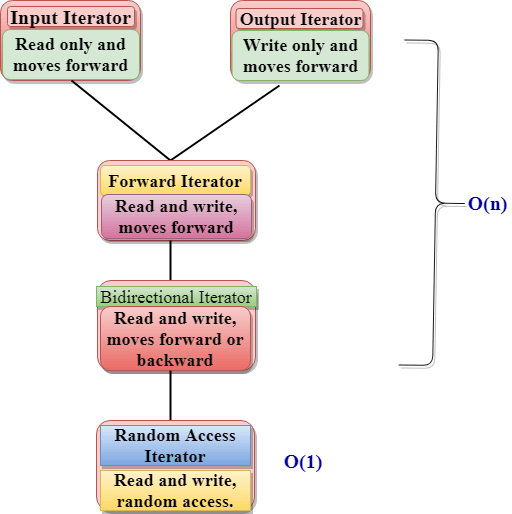
C Iterators Javatpoint
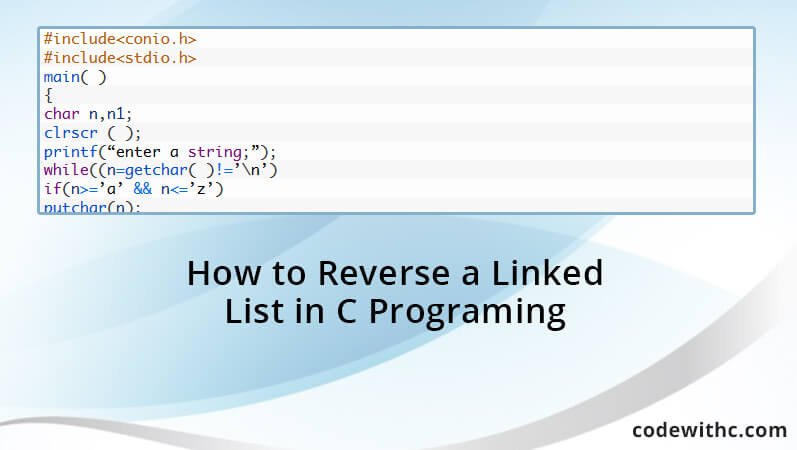
How To Reverse Linked List Using Iteration In C Programming Code With C
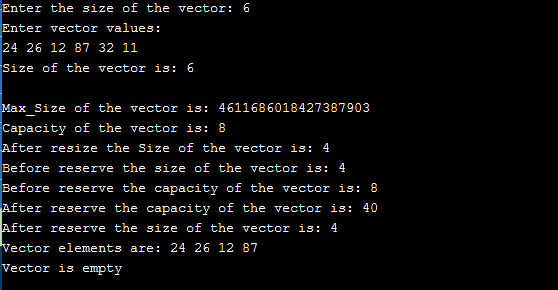
C Vector Example Vector In C Tutorial
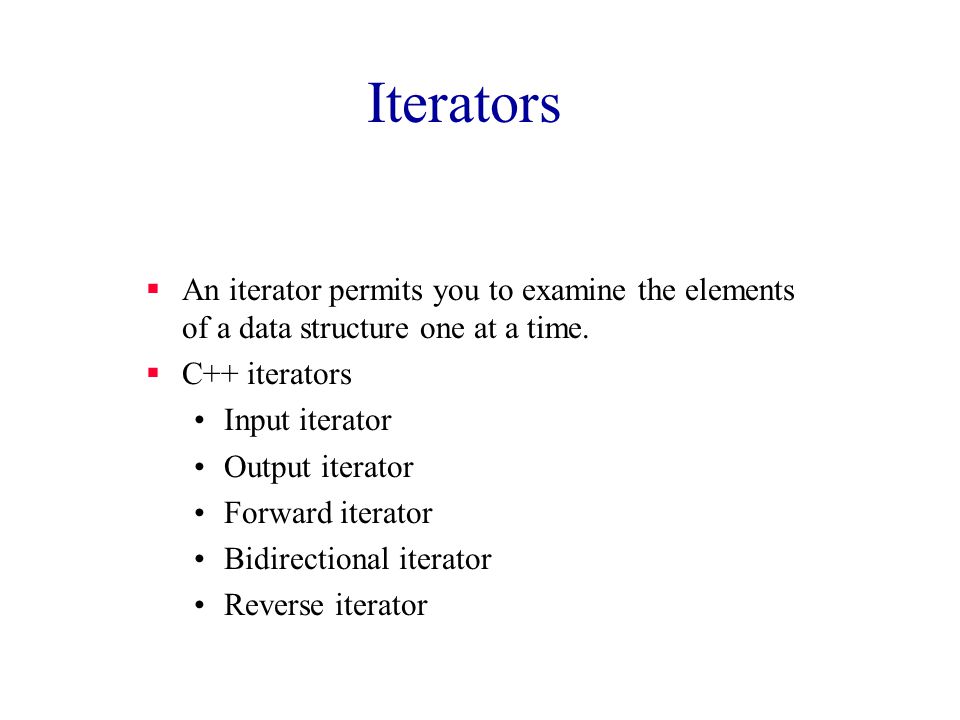
Iterators Chain Variants Iterators An Iterator Permits You To Examine The Elements Of A Data Structure One At A Time C Iterators Input Iterator Ppt Download
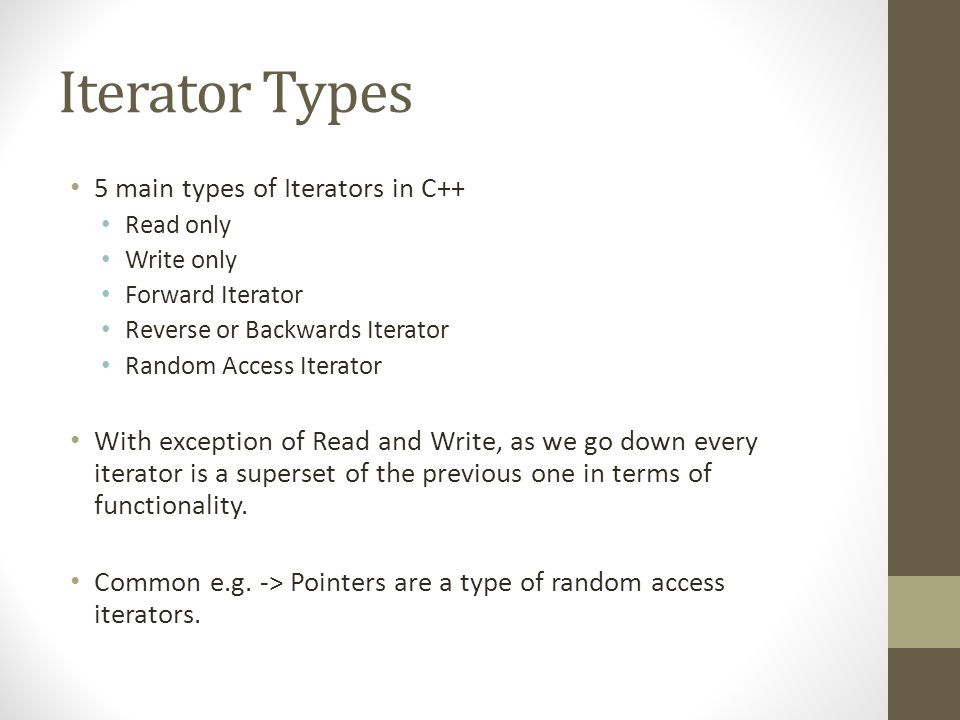
Iterators Iterator An Iterator In C Is A Concept That Refines The Iterator Design Pattern Into A Specific Set Of Behaviors That Work Well With The Ppt Download
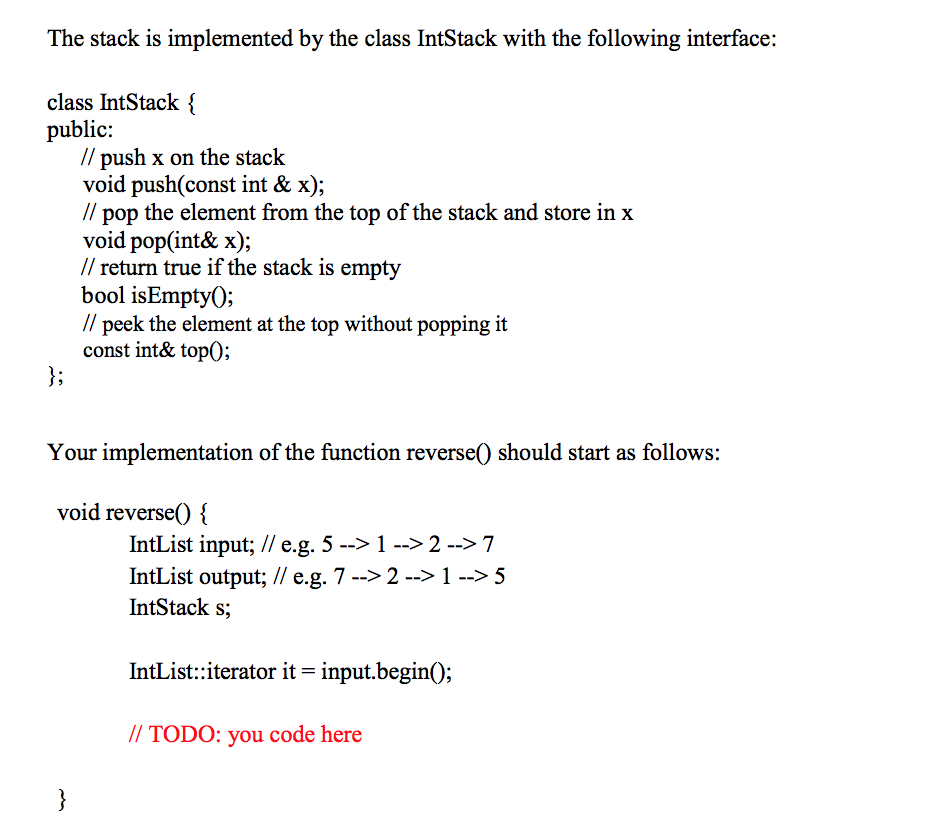
Solved Problem 2 25 Points Write The Function Reverse Chegg Com
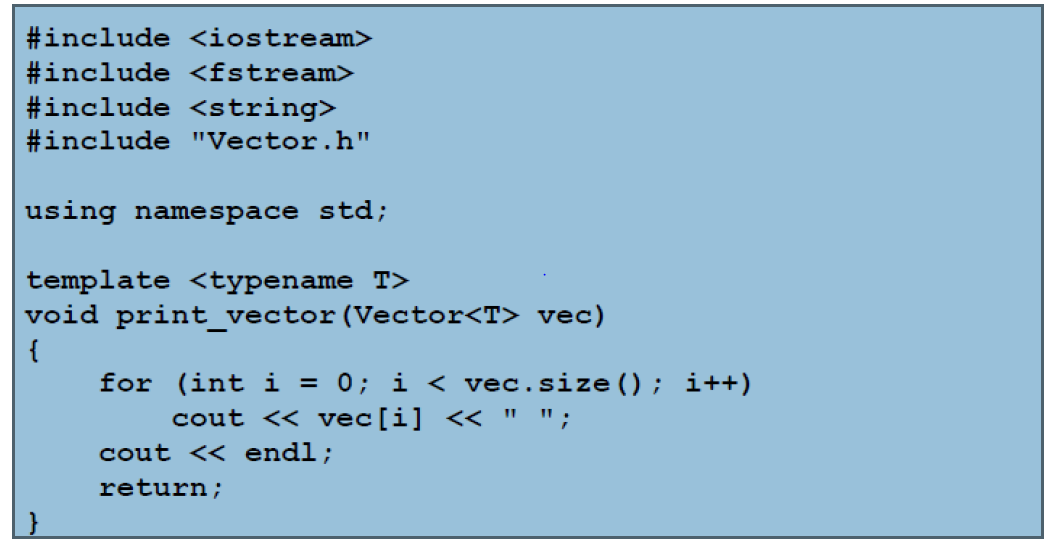
Solved Please Use C Please Help Thank You So Much Ad Chegg Com
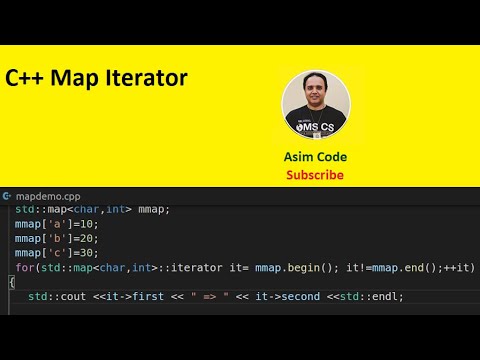
C Reverse Iterators Youtube
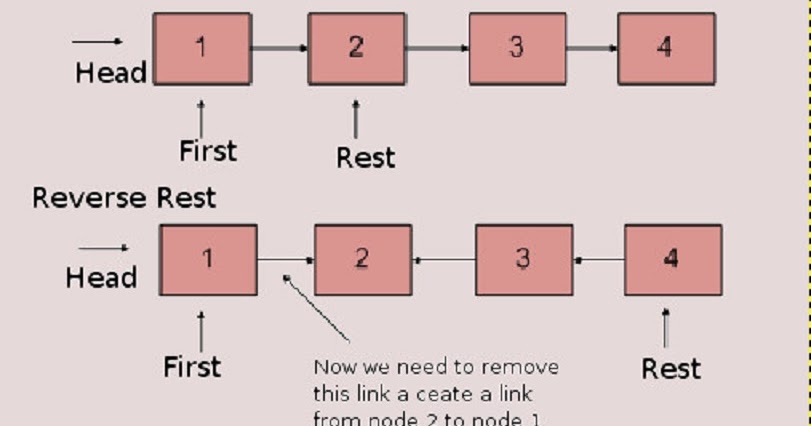
Javarevisited How To Reverse A Linked List In Java Using Recursion And Iteration Loop Example

C Vector Example Vector In C Tutorial
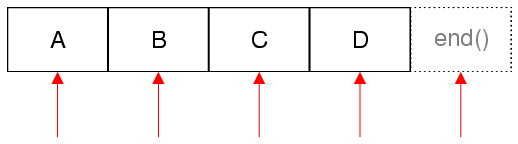
Container Classes Qt Core 5 15 1

Apostila Programacao Orientada A Objeto Com C Stl Referencia Programacao Orientada Docsity
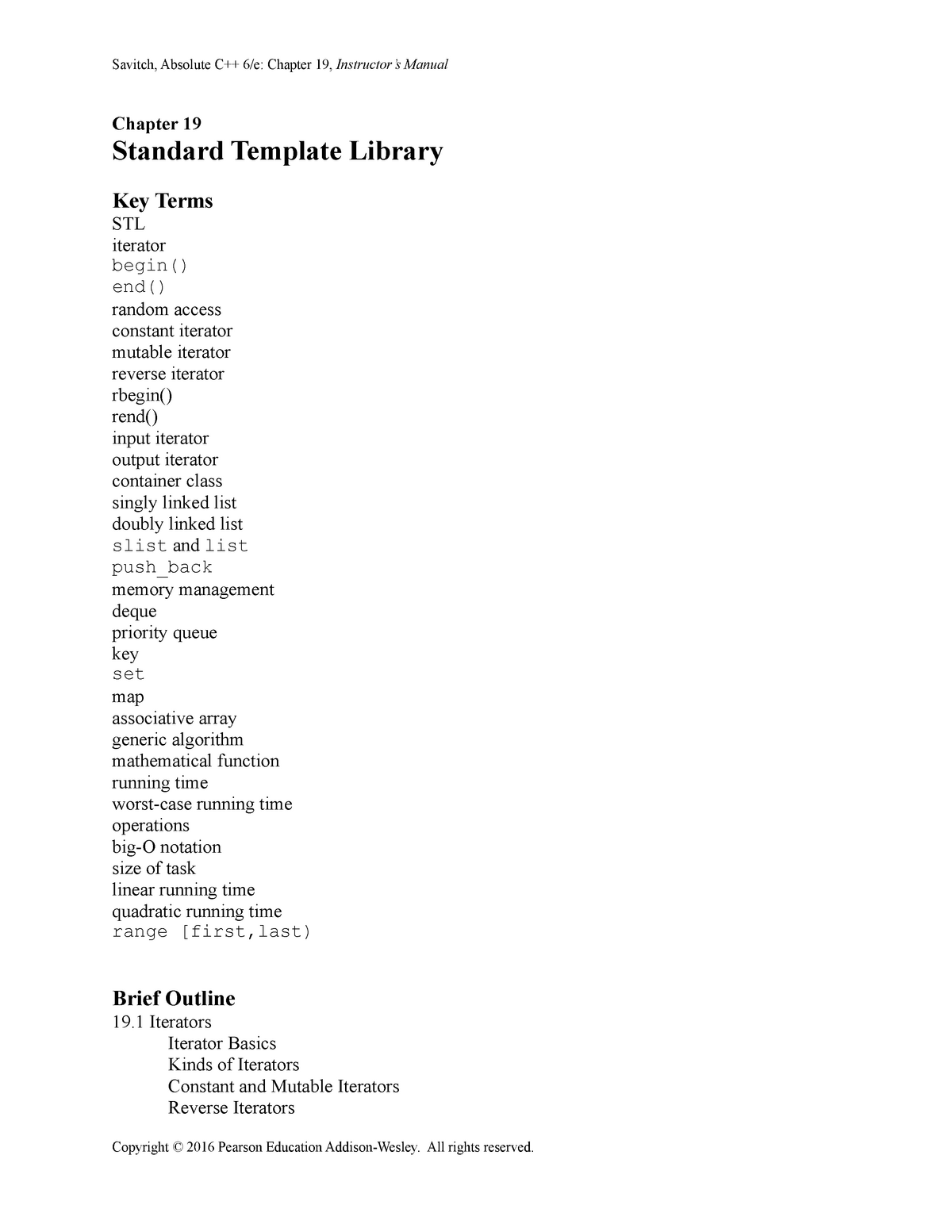
Chapter 19 Studocu
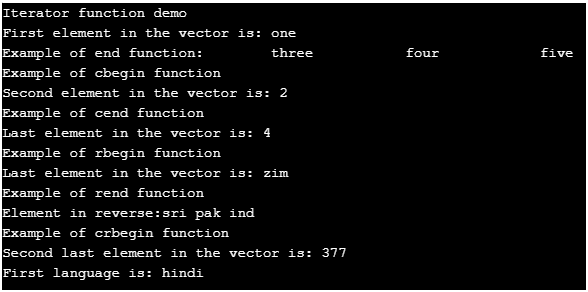
C Vector Functions Laptrinhx
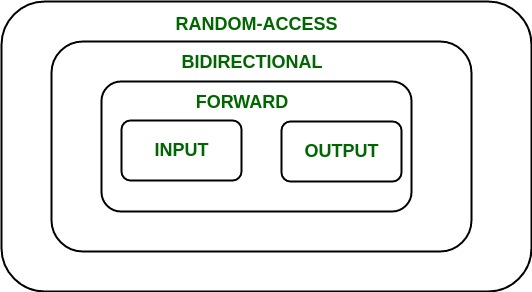
Introduction To Iterators In C Geeksforgeeks
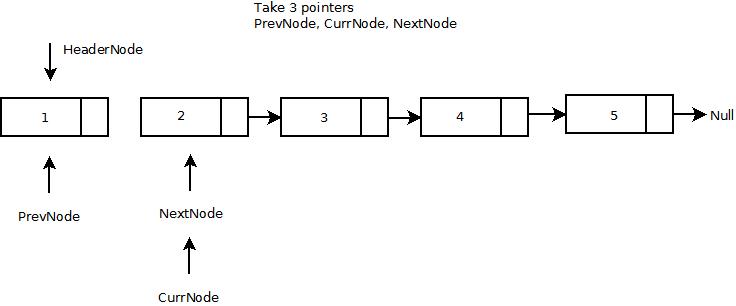
Reverse A Single Linked List Iterative Procedure Programming Interview Questions
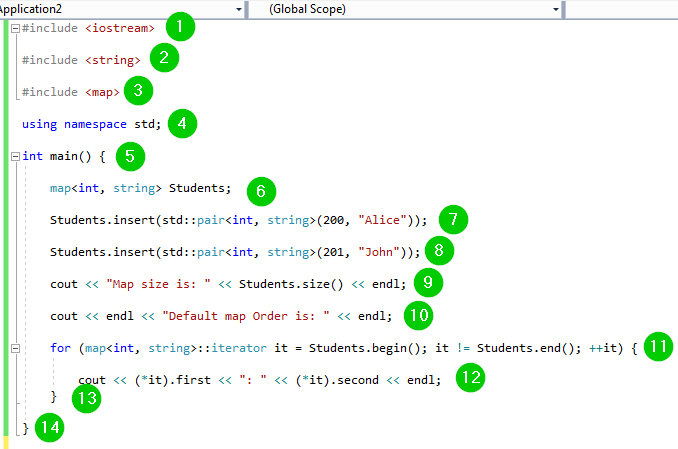
Map In C Standard Template Library Stl With Example

Stl Container For C Knowledge Sharing Simple Application Of Set Container And Map Container Programmer Sought
Iterators Json For Modern C
Code Race C Program To Reverse A Number
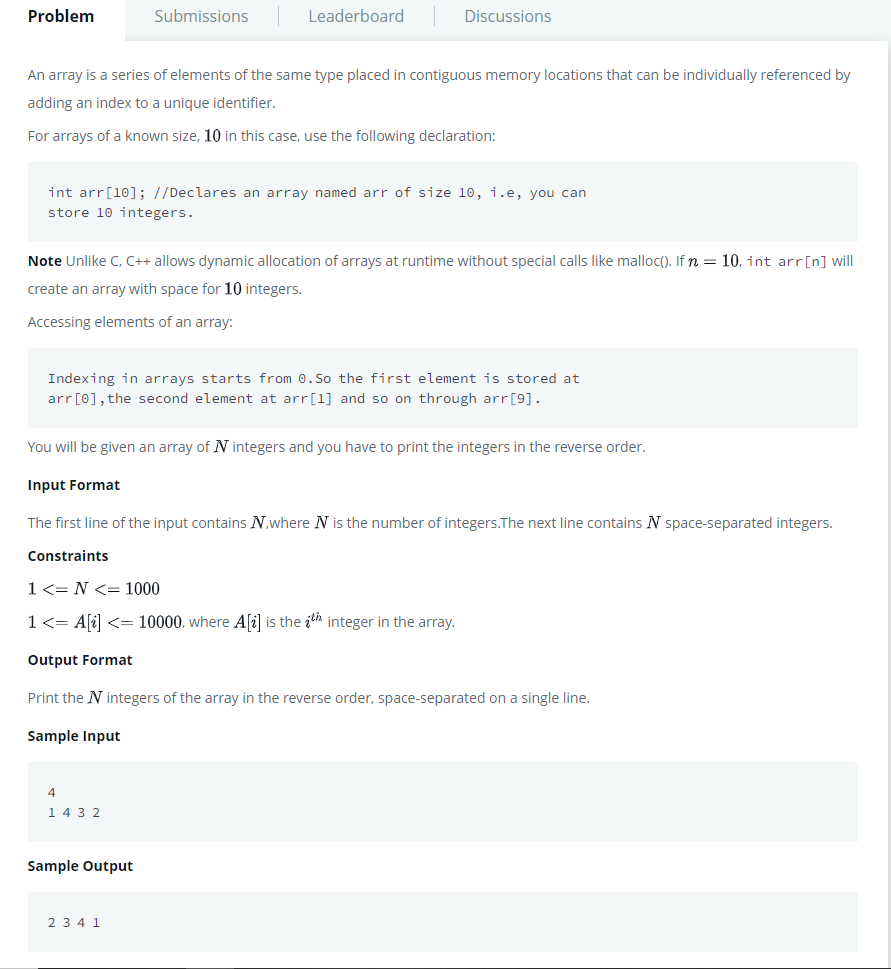
Solved C You Must Use A Vector You May Not Use Rev Chegg Com
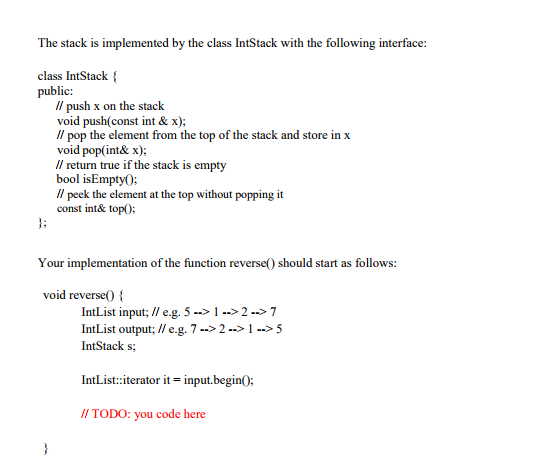
Problem 2 25 Points Write The Function Reverse0 Chegg Com
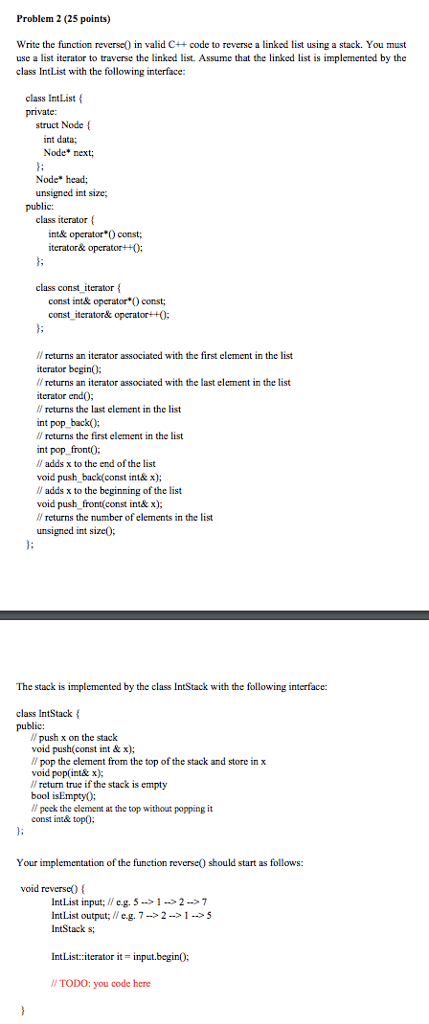
Solved Problem 2 25 Points Write The Function Reverse0 Chegg Com

Garbage Value Of Iterator Value In C Stack Overflow
One Way To Reverse Copy A Deque Deque Data Structure C

Python Reversed Method And Usage Explaned With Examples
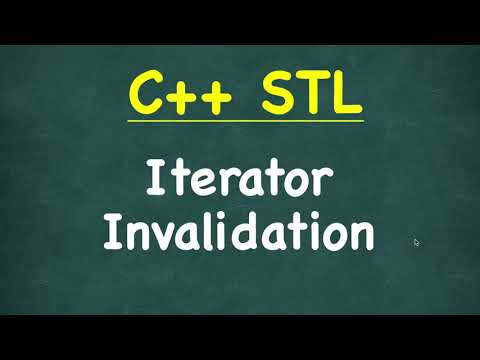
Iterator Invalidation C Stl Standard Template Library Youtube

C Std Array Declare Initialize Passing Std Array To Function
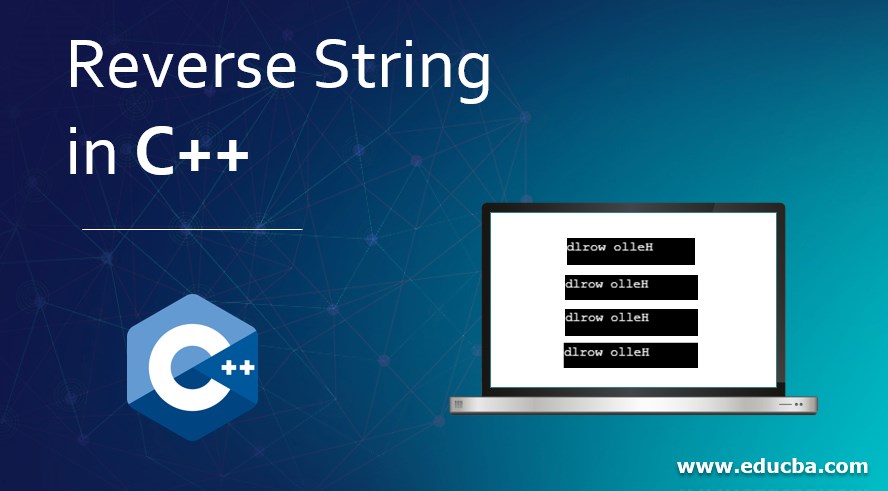
Reverse String In C How To Implement Reverse String In C

Visual Studio C Reverse Iterator Compiles But Throws An Error At Runtime Stack Overflow
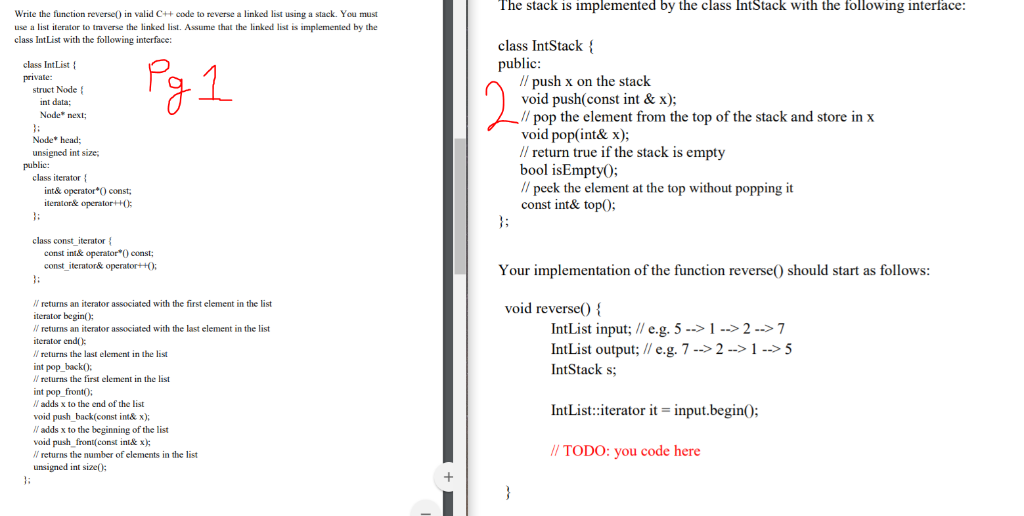
Solved The Stack Is Implemented By The Class Intstack Wit Chegg Com
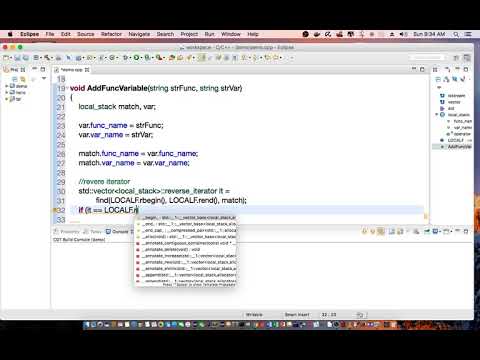
C Reverse Iterator Tutorial Youtube
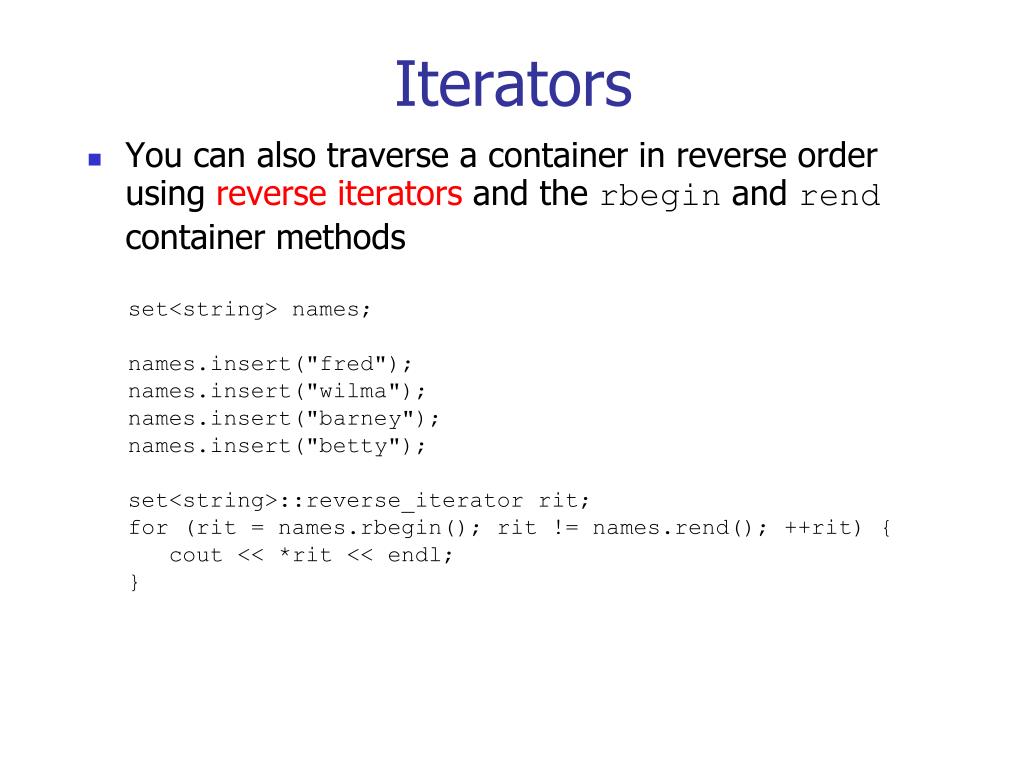
Ppt Standard Template Library Stl Powerpoint Presentation Free Download Id

Reverse A List In Python Five Techniques With Examples
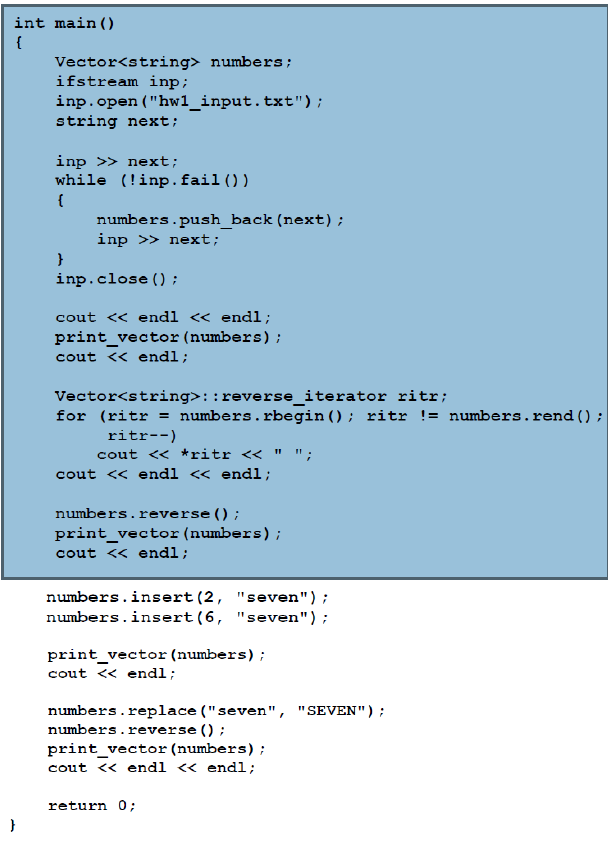
Solved Please Use C Please Help Thank You So Much Ad Chegg Com
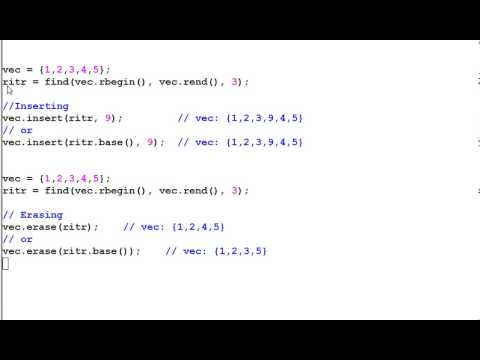
Learn Stl Tricky Reverse Iterator Youtube
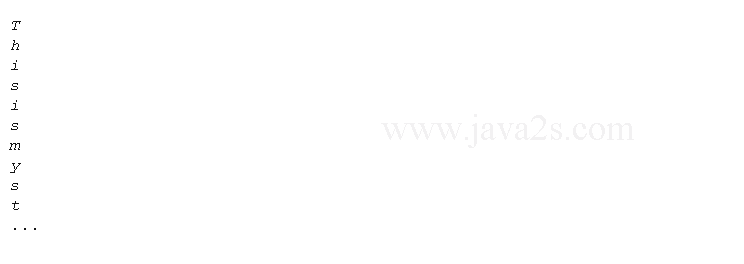
String Reverse Iterator With Rbegin And Rend Const Iterators C Stl

The C Standard Template Library Stl Programming On Iterators Algorithm And Containers Tutorials With C Program Examples
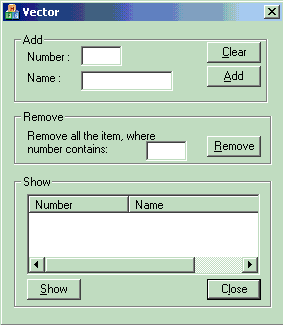
A Study Of Stl Container Iterator And Predicates Codeproject

C Std Array Declare Initialize Passing Std Array To Function

C Program To Reverse A String

The Difference Between Std Copy Backward And Std Copy With Reverse Iterators Fluent C
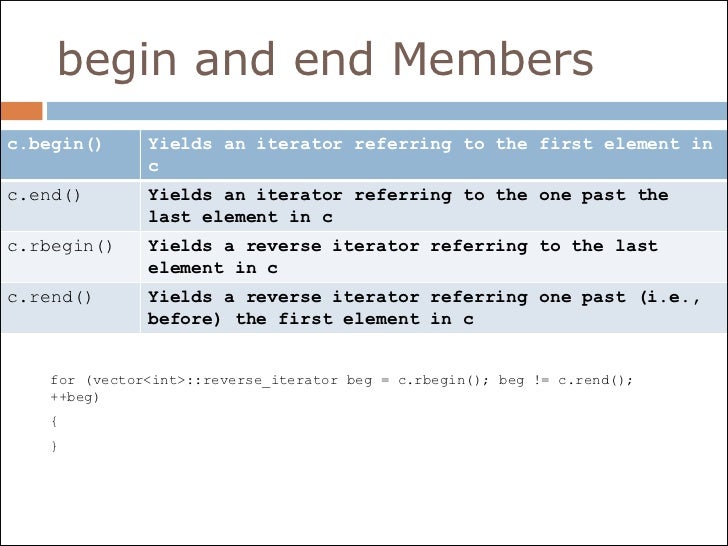
An Introduction To Part Of C Stl
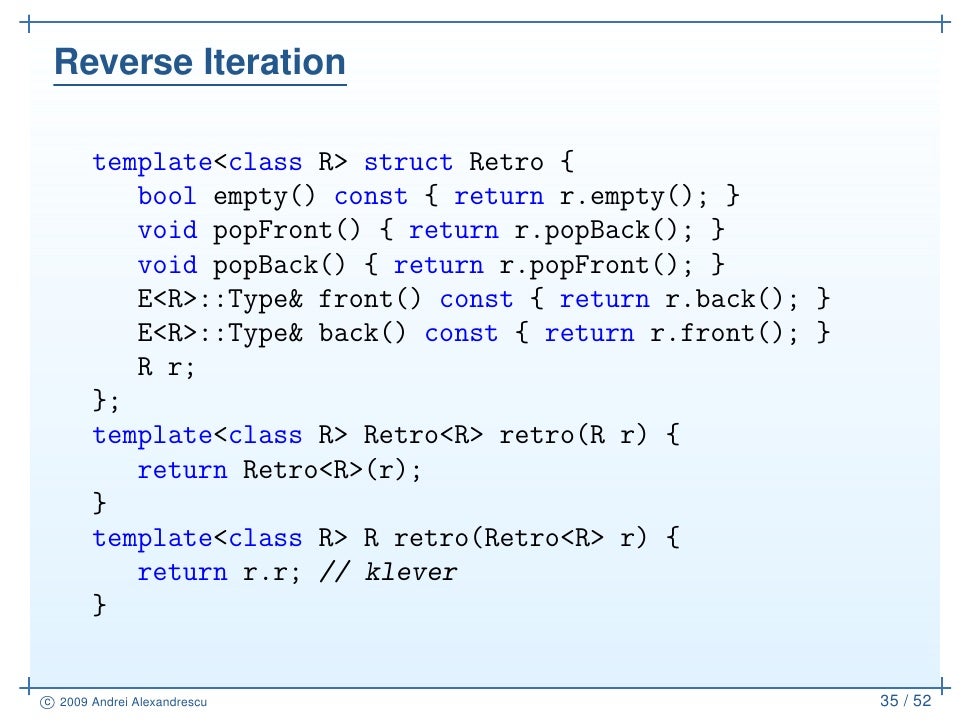
Iterators Must Go
Learning C The Stl And Iterators By Michael Mcmillan Level Up Coding

Inherit All Functions From User Defined Iterator In Reverse Iterator Stack Overflow

Bidirectional Iterators In C Geeksforgeeks
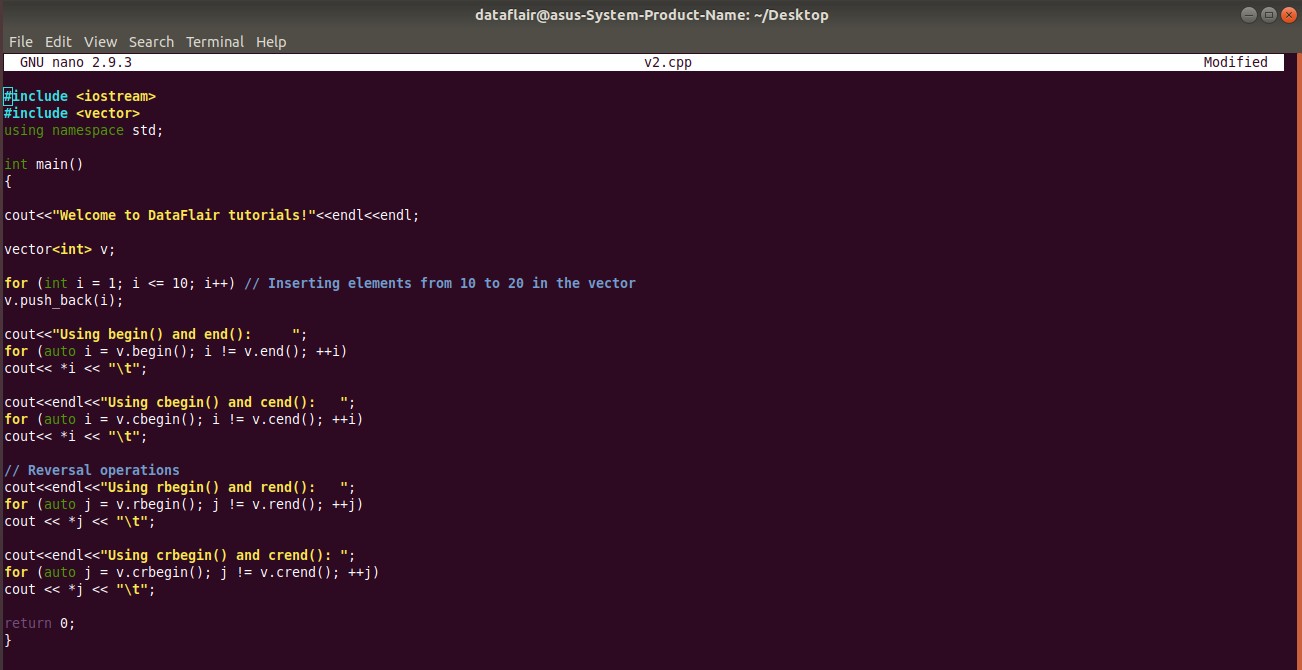
C Vector Learn 5 Types Of Functions Associated With Vector Dataflair
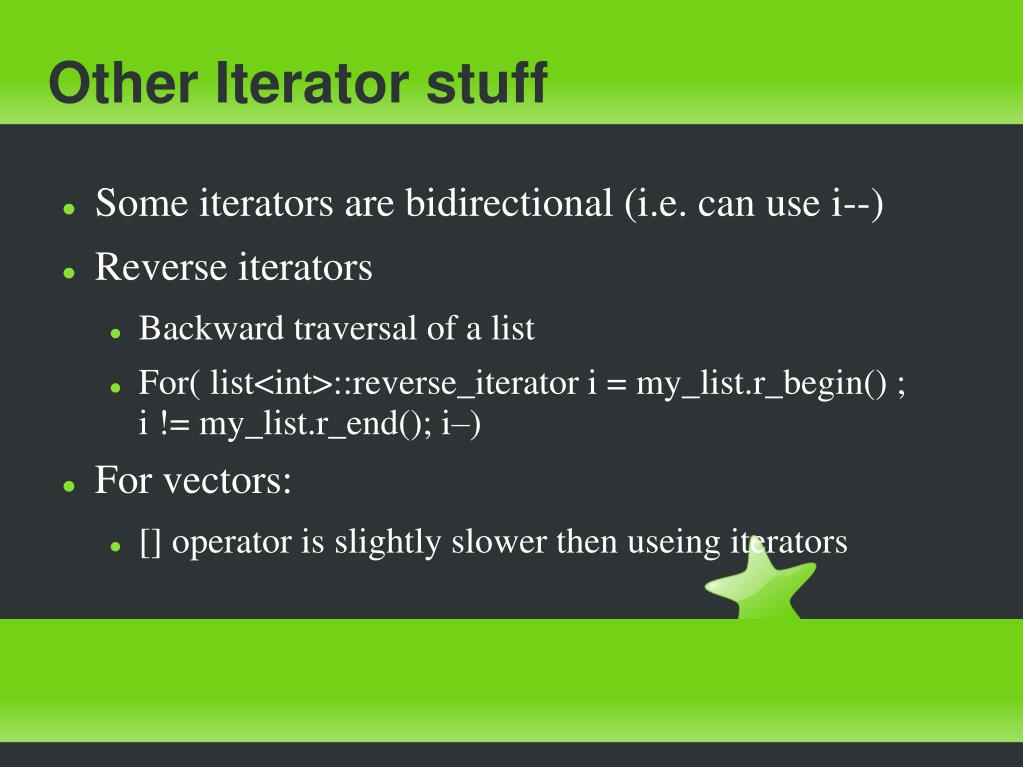
Ppt Standard Template Library Stl Powerpoint Presentation Free Download Id